Practical Applications of Generative Fill
No need for props to shoot the product photo. Add complementary ingredients or elements to product photos digitally to improve aesthetic appeal.
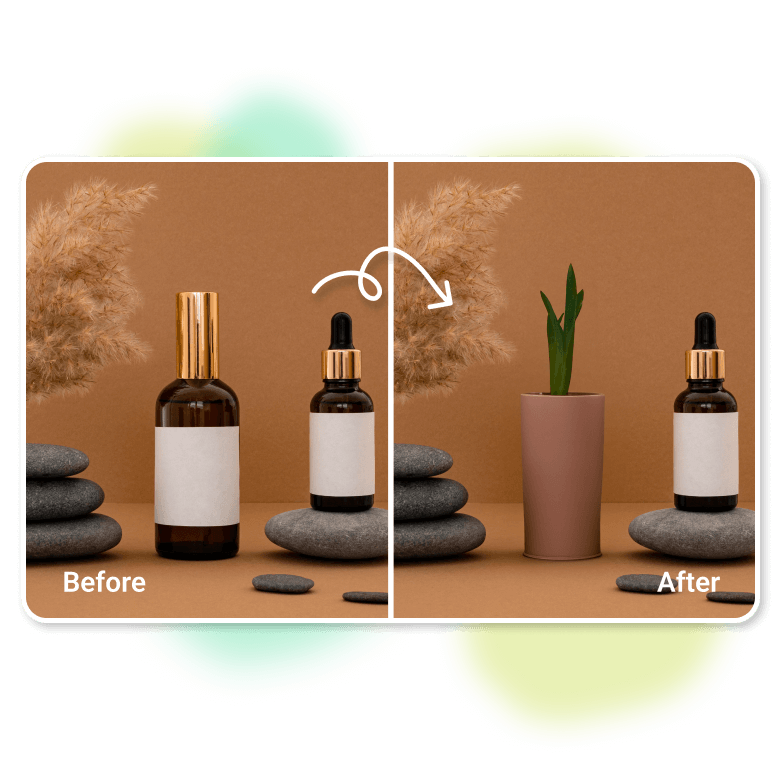
import requests
url = 'https://api.lightxeditor.com/external/api/v1/replace'
headers = {
'Content-Type': 'application/json',
'x-api-key': '<Insert your API Key>' # Replace with your actual API key
}
data = {
"imageUrl": "https://example.com/your-image.jpg", # Replace with the URL of your input image
"maskedImageUrl": "https://example.com/your-masked-image.jpg", # Replace with the URL of your input masked image
"textPrompt": "YourInputPrompt" # Replace with your specific input prompt
}
response = requests.post(url, headers=headers, json=data)
# Check if the request was successful
if response.status_code == 200:
print("Request was successful!")
print(response.json())
else:
print(f"Request failed with status code: {response.status_code}")
print(response.text)
const fetch = require('node-fetch'); // Only needed if you are using Node.js
const url = 'https://api.lightxeditor.com/external/api/v1/replace';
const apiKey = '<Insert your API Key>'; // Replace with your actual API key
const data = {
"imageUrl": "https://example.com/your-image.jpg", // Replace with the URL of your input image
"maskedImageUrl": "https://example.com/your-masked-image.jpg", // Replace with the URL of your input masked image
"textPrompt": "YourInputPrompt" // Replace with your specific input prompt
};
const options = {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'x-api-key': apiKey
},
body: JSON.stringify(data)
};
fetch(url, options)
.then(response => {
if (!response.ok) {
throw new Error(`Request failed with status code ${response.status}`);
}
return response.json();
})
.then(data => {
console.log('Request was successful!');
console.log(data);
})
.catch(error => {
console.error('Error:', error);
});
}
import Foundation
// Define the URL and API key
let url = URL(string: "https://api.lightxeditor.com/external/api/v1/replace")!
let apiKey = "<Insert your API Key>" // Replace with your actual API key
// Define the request body
let requestBody: [String: Any] = [
"imageUrl": "https://example.com/your-image.jpg", // Replace with the URL of your input image
"maskedImageUrl": "https://example.com/your-masked-image.jpg", // Replace with the URL of your input masked image
"textPrompt": "YourInputPrompt" // Replace with your specific input prompt
]
// Convert request body to JSON data
let jsonData = try! JSONSerialization.data(withJSONObject: requestBody, options: [])
// Create the URLRequest object
var request = URLRequest(url: url)
request.httpMethod = "POST"
request.setValue("application/json", forHTTPHeaderField: "Content-Type")
request.setValue(apiKey, forHTTPHeaderField: "x-api-key")
request.httpBody = jsonData
// Create the URLSession data task
let task = URLSession.shared.dataTask(with: request) { data, response, error in
// Handle the response
if let error = error {
print("Error: \(error)")
return
}
guard let httpResponse = response as? HTTPURLResponse, (200...299).contains(httpResponse.statusCode) else {
print("Unexpected response")
return
}
if let data = data {
do {
// Parse and print the JSON response
let jsonResponse = try JSONSerialization.jsonObject(with: data, options: [])
print("Response: \(jsonResponse)")
} catch {
print("Error parsing JSON: \(error)")
}
}
}
// Start the task
task.resume()
import okhttp3.MediaType.Companion.toMediaType
import okhttp3.OkHttpClient
import okhttp3.Request
import okhttp3.RequestBody.Companion.toRequestBody
import okhttp3.Response
import org.json.JSONObject
fun main() {
// Define the URL and API key
val url = "https://api.lightxeditor.com/external/api/v1/replace"
val apiKey = "<Insert your API Key>" // Replace with your actual API key
// Define the request body
val requestBody = JSONObject().apply {
put("imageUrl", "https://example.com/your-image.jpg") // Replace with the URL of your input image
put("maskedImageUrl", "https://example.com/your-masked-image.jpg") // Replace with the URL of your input masked image
put("textPrompt", "YourInputPrompt") // Replace with your specific input prompt
}.toString()
// Create OkHttpClient
val client = OkHttpClient()
// Create the request
val request = Request.Builder()
.url(url)
.post(requestBody.toRequestBody("application/json; charset=utf-8".toMediaType()))
.addHeader("x-api-key", apiKey)
.build()
// Make the request
client.newCall(request).execute().use { response ->
if (!response.isSuccessful) {
throw IOException("Unexpected code $response")
}
// Print the response
val responseData = response.body?.string()
println("Response: $responseData")
}
}
Method- Post
curl --location 'https://api.lightxeditor.com/external/api/v1/replace' \
--header 'Content-Type: application/json' \
--header 'x-api-key: <Insert your API Key>' \
--data '{
"imageUrl": "https://example.com/your-image.jpg", // Replace with the URL of your input image
"maskedImageUrl": "https://example.com/your-masked-image.jpg", // Replace with the URL of your input masked image
"textPrompt": "YourInputPrompt" // Replace with your specific input prompt
}'
Choose Your Plan
Explore a variety of pricing plans designed to meet your unique requirements. Find the perfect fit and start today.
Prompt-Based Object Placement
A key feature of the tool is its integration with prompt-based inputs, giving you complete creative freedom to swap objects using simple text prompts. Just describe the element you want to generate, and the tool ensures it blends seamlessly with the rest of the image.
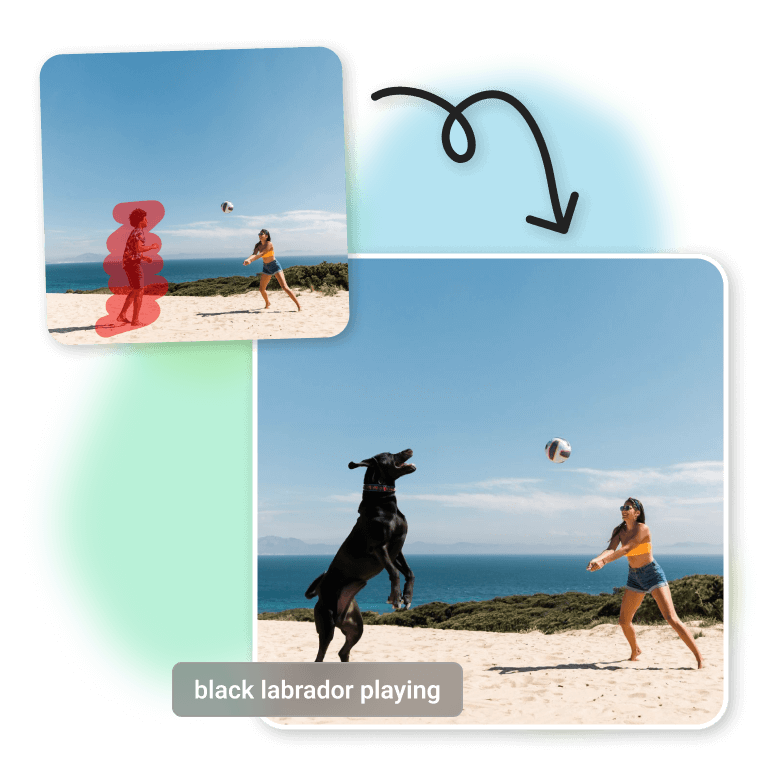
Smart Context Analysis
The tool detects the image context to understand surrounding elements for accurate replacements. When creating or replacing objects in image, the API analyzes textures, colors, and edges properly. It ensures everything looks contextual and doesn't appear out of place.
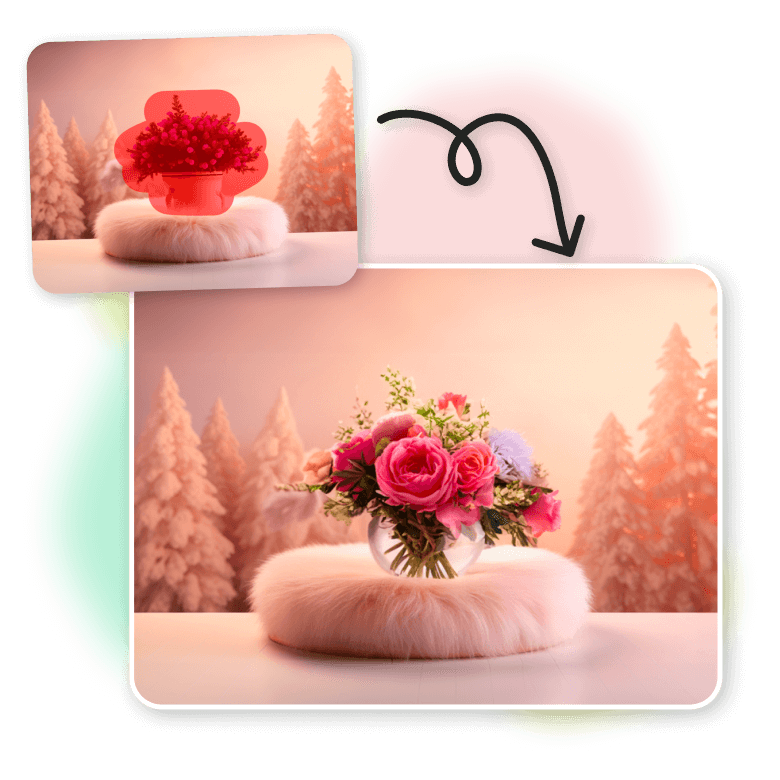
Perfect Object Scaling and Proportion
The tool maintains proper size ratios and perspective relative to other objects in the image. For example, when adding a tree to a photo with a person, it ensures the tree’s size is proportionate and realistic.
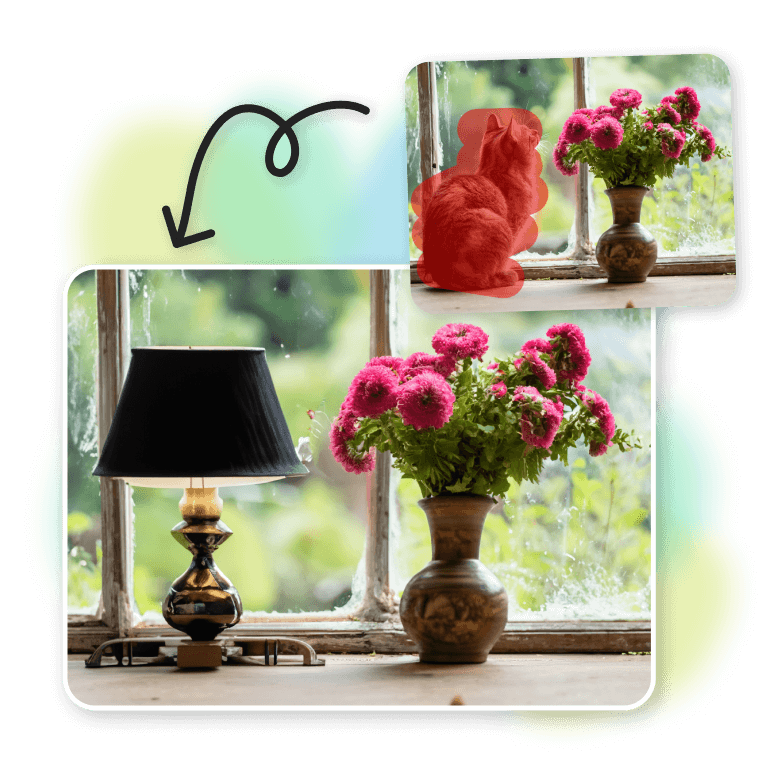
Prompt-Based Object Replacer API
A key feature of the tool is its integration with prompt-based inputs. You have complete creative freedom to design what you envision. The tool ensures whatever you create blends smoothly with the rest of the image.
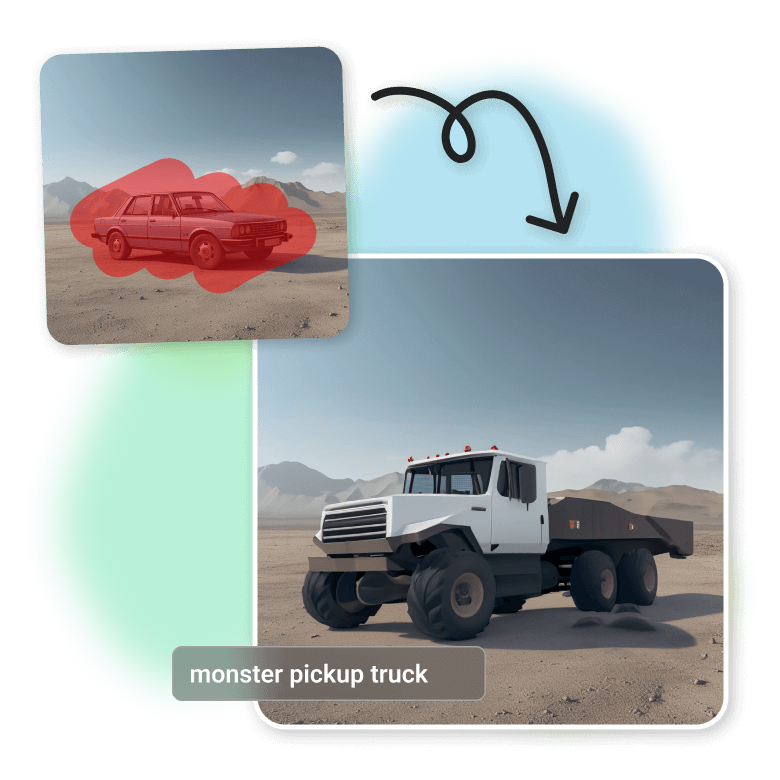
Start with Free Credits
Get started with LightX APIs today! Receive 25 free credits instantly on API signup – no credit card required
Frequently Asked Questions
Our generative fill is a very straightforward—masking and prompting tool. Simply upload your image, mask the area where you'd like the new object, and use a text prompt to describe the object. The AI will then generate and seamlessly integrate it into the image.
Still Unsure? Allow Us To Assist You!
Write to us and we'd love to understand your requirements