AI Image Upscaler API
Easy-to-integrate LightX Upscale API automatically enhances user-uploaded images — without any manual work on the backend. Let users upload low-quality images on your website or app, and they’ll be instantly transformed into high-quality visuals on your platform.
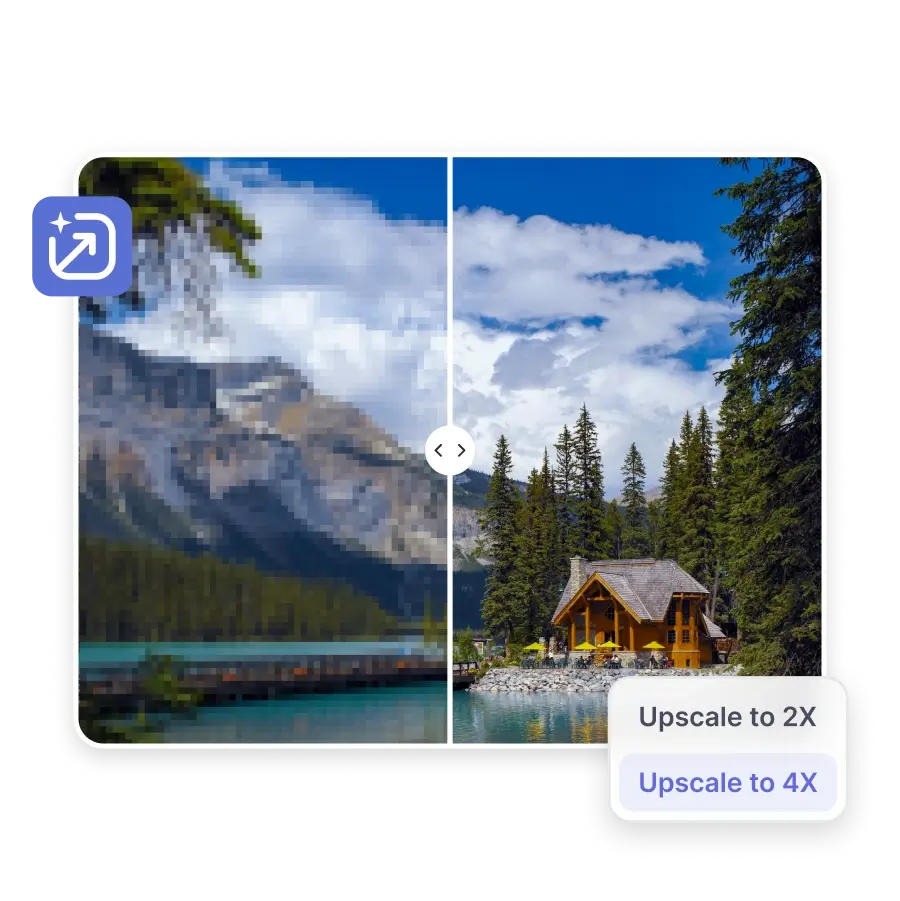
Ideal users of the upscaler API
Your seller-uploaded low-resolution product photos will automatically become high-quality, so they don't pixelate when a buyer zooms in.
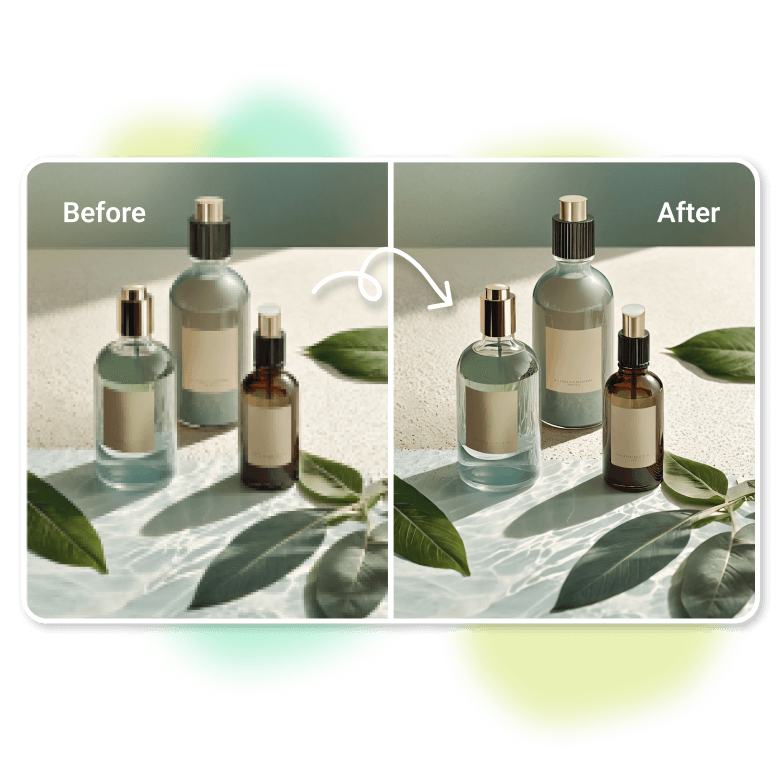
import requests
url = "https://api.lightxeditor.com/external/api/v2/upscale/"
headers = {
"Content-Type": "application/json",
"x-api-key": "<enter x-api-key>"
}
data = {
"imageUrl": "imageUrl",
"quality": 2 # 2X or 4X only
}
response = requests.post(url, json=data, headers=headers)
print(response.json())
# Check if the request was successful
if response.status_code == 200:
print("Request was successful!")
print(response.json())
else:
print(f"Request failed with status code: {response.status_code}")
print(response.text)
const fetch = require('node-fetch'); // Only needed if you are using Node.js
const url = 'https://api.lightxeditor.com/external/api/v2/upscale/';
const headers = {
'Content-Type': 'application/json',
'x-api-key': '<enter x-api-key>'
};
const data = {
"imageUrl": "imageUrl",
"quality": 2 # 2X or 4X only
};
fetch(url, {
method: 'POST',
headers: headers,
body: JSON.stringify(data)
})
.then(response => response.json())
.then(data => console.log('Response:', data))
.catch(error => console.error('Error:', error));
}
import Foundation
let url = URL(string: "https://api.lightxeditor.com/external/api/v2/upscale/")!
var request = URLRequest(url: url)
request.httpMethod = "POST"
request.addValue("application/json", forHTTPHeaderField: "Content-Type")
request.addValue("<enter x-api-key>", forHTTPHeaderField: "x-api-key")
let body: [String: Any] = [
"imageUrl": "imageUrl",
"quality": 2 // 2X or 4X only
]
do {
let jsonData = try JSONSerialization.data(withJSONObject: body, options: [])
request.httpBody = jsonData
} catch {
print("Error serializing JSON: \(error)")
return
}
let task = URLSession.shared.dataTask(with: request) { data, response, error in
if let error = error {
print("Error: \(error)")
return
}
guard let data = data else {
print("No data received.")
return
}
do {
let jsonResponse = try JSONSerialization.jsonObject(with: data, options: [])
print("Response: \(jsonResponse)")
} catch {
print("Error parsing response: \(error)")
}
}
task.resume()
import Foundation
let url = URL(string: "https://api.lightxeditor.com/external/api/v2/upscale/")!
var request = URLRequest(url: url)
request.httpMethod = "POST"
request.addValue("application/json", forHTTPHeaderField: "Content-Type")
request.addValue("<enter x-api-key>", forHTTPHeaderField: "x-api-key")
let body: [String: Any] = [
"imageUrl": "imageUrl",
"quality": 2 // 2X or 4X only
]
do {
let jsonData = try JSONSerialization.data(withJSONObject: body, options: [])
request.httpBody = jsonData
} catch {
print("Error serializing JSON: \(error)")
return
}
let task = URLSession.shared.dataTask(with: request) { data, response, error in
if let error = error {
print("Error: \(error)")
return
}
guard let data = data else {
print("No data received.")
return
}
do {
let jsonResponse = try JSONSerialization.jsonObject(with: data, options: [])
print("Response: \(jsonResponse)")
} catch {
print("Error parsing response: \(error)")
}
}
task.resume()
Method- Post
curl --location 'https://api.lightxeditor.com/external/api/v2/upscale/' \
--header 'Content-Type: application/json' \
--header 'x-api-key: <enter x-api-key>' \
--data '{
"imageUrl": "imageUrl",
"quality": 2 # 2X or 4X only
}'
Choose Your Plan
Explore a variety of pricing plans designed to meet your unique requirements. Find the perfect fit and start today.
Upscale photos up to 4x
LightX AI Image Quality Enhancer can make images 4K. You don’t need to manually edit or replace low-quality images. Just integrate our API, and it will automatically enhance image quality to 2x or 4x wherever and whenever you need it. This saves you time on editing and cuts costs by reducing the need for reshoots.
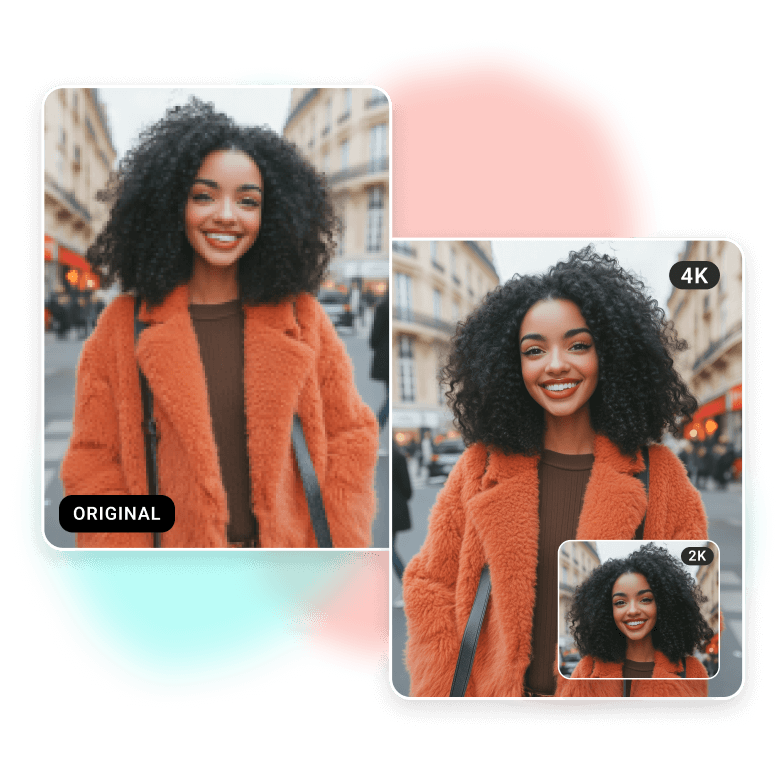
Process large volumes of images quickly
Get rapid results — your images will be upscaled by the time your webpage loads. The tool maintains the original image quality, colors, and structure — it doesn’t alter the processed image details; it simply enhances the clarity and increases the resolution. Whether you need to upscale faces, text, products, or landscapes, the LightX Upscaler will make them better, sharper, and larger.
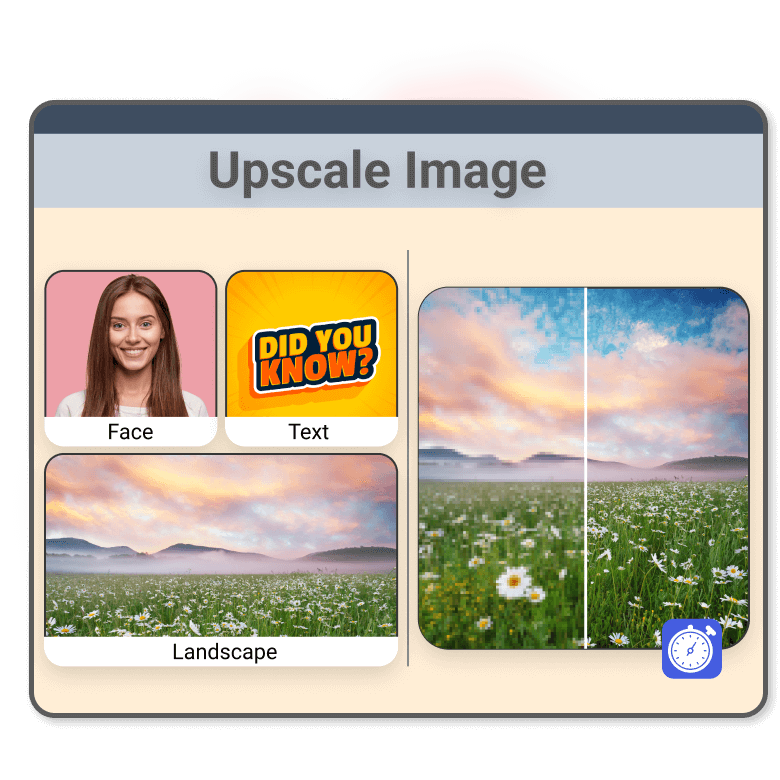
100% data privacy & security
With reliable security measures, including data encryption in transit and at rest, LightX ensures your data stays protected. Your images are not used for training our models and are automatically deleted 24 hours after processing.
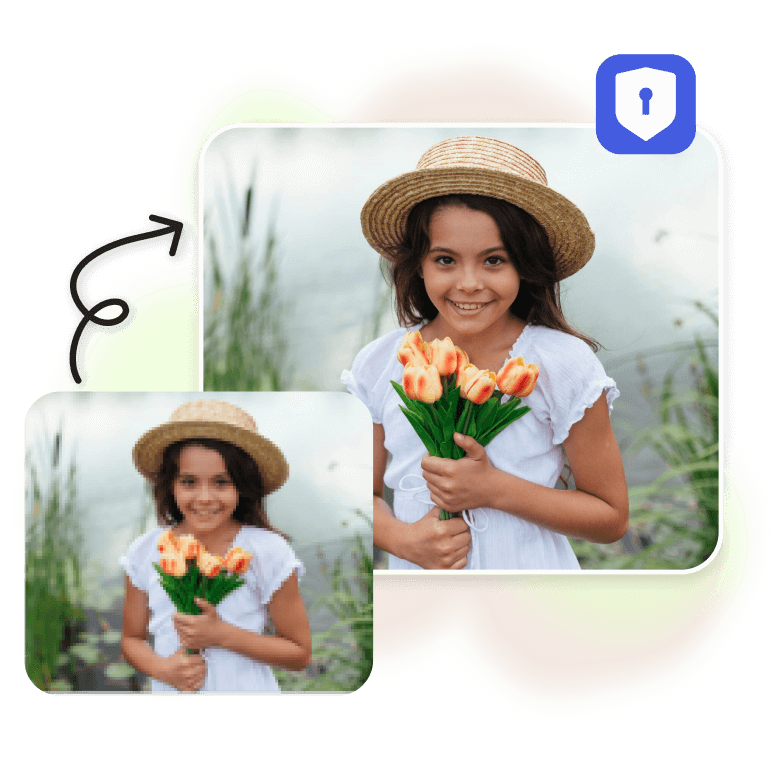
How to get started?
It’s super easy and straightforward!
1. Click the “Generate API Key” button below.
2. You’ll be prompted to sign in or sign up if you haven't already.
3. After signing in, fill in a few quick details.
4. Once done, you’ll instantly receive 25 free credits along with your API key.
5. Head over to our API Documentation for end-to-end integration instructions.
6. Visit the API Pricing page if you need more credits later.
7. Still have questions? Our support team is happy to help anytime.
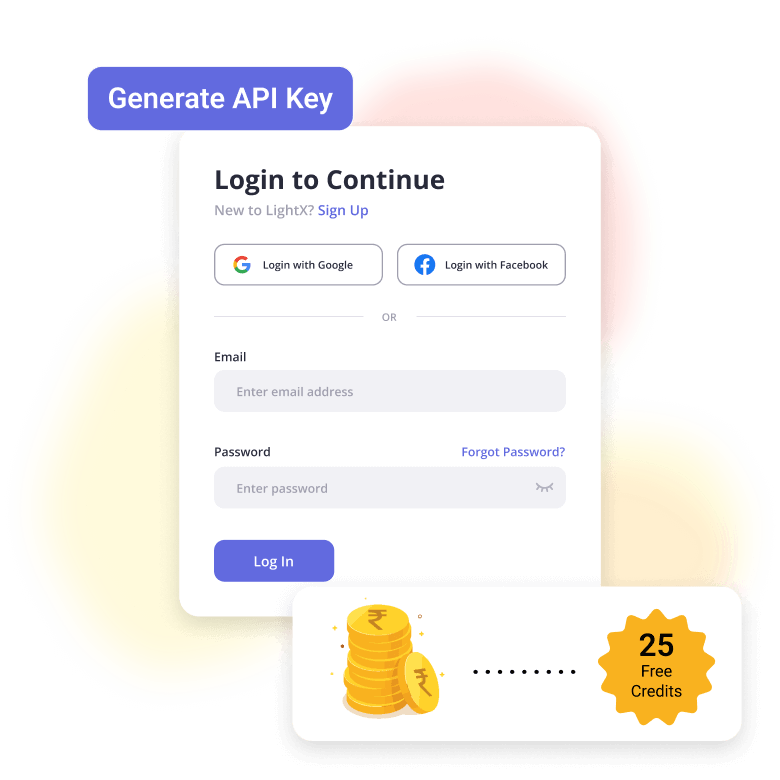
Before you integrate: Is the API right for you?
If you're a one-time user or a business that only needs to upscale one or two images occasionally, our upscaling tool is a more convenient and cost-effective option. It's available on both iOS and Android, so you can upscale your photos anytime, anywhere.
Businesses looking to try before they buy can receive 25 free credits to test the Image Upscaler API upon sign-up.
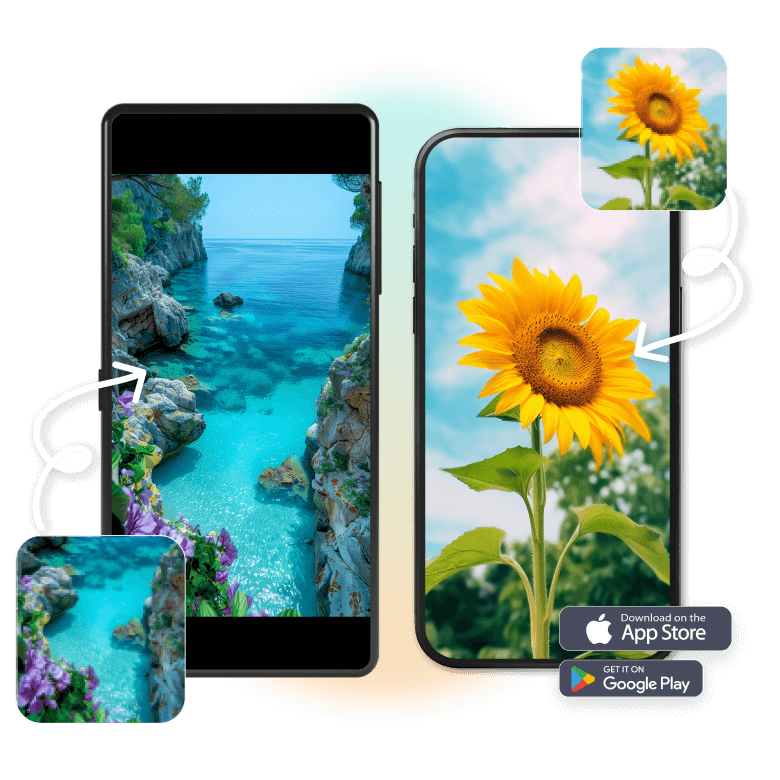
Start with Free Credits
Get started with LightX APIs today! Receive 25 free credits instantly on API signup – no credit card required
Frequently Asked Questions
When you send a low-resolution image to the LightX Upscale API, the image is securely uploaded to our server. It is then processed and turned into a high-resolution image, increasing its size and enhancing its quality. The API returns a high-resolution version of the image, ready to be used on your website or app or for printing. This entire process is automatic and takes only a few seconds.
Still Unsure? Allow Us To Assist You!
Write to us and we'd love to understand your requirements